python中的st什么意思
Python中的st是什么意思?
在Python中,st通常指的是字符串(string)。字符串是一種數據類型,用于表示文本或字符序列。在Python中,字符串是不可變的,也就是說,一旦創建了一個字符串,就不能修改它的值。
字符串在Python中非常常用,可以用于各種操作,比如文本處理、數據解析、網絡編程等等。Python提供了豐富的字符串處理函數和方法,可以方便地對字符串進行操作和處理。
Python中的字符串是如何表示的?
Python中的字符串可以用單引號(')或雙引號(")括起來表示,例如:
s1 = 'hello'
s2 = "world"
Python還支持三引號('''或""")表示多行字符串,例如:
s3 = '''This is a
multi-line
string.'''
三引號表示的字符串可以包含換行符和其他特殊字符,非常方便。
Python中的字符串有哪些常用操作?
Python中的字符串支持許多常用操作,例如:
- 字符串拼接:使用加號(+)將兩個字符串拼接起來,例如:
s1 = 'hello'
s2 = 'world'
s3 = s1 + ' ' + s2
print(s3) # 輸出:hello world
- 字符串索引:可以通過索引訪問字符串中的單個字符,索引從0開始,例如:
s = 'hello'
print(s[0]) # 輸出:h
print(s[1]) # 輸出:e
print(s[-1]) # 輸出:o(-1表示最后一個字符)
- 字符串切片:可以通過切片操作訪問字符串中的子串,例如:
s = 'hello world'
print(s[0:5]) # 輸出:hello
print(s[6:]) # 輸出:world
print(s[:5]) # 輸出:hello
- 字符串長度:可以使用len函數獲取字符串的長度,例如:
s = 'hello'
print(len(s)) # 輸出:5
- 字符串查找:可以使用find方法查找字符串中是否包含某個子串,例如:
s = 'hello world'
print(s.find('world')) # 輸出:6
print(s.find('python')) # 輸出:-1(表示沒找到)
- 字符串替換:可以使用replace方法替換字符串中的某個子串,例如:
s = 'hello world'
s = s.replace('world', 'python')
print(s) # 輸出:hello python
- 字符串分割:可以使用split方法將字符串按照某個分隔符分割成多個子串,例如:
s = 'hello,world'
print(s.split(',')) # 輸出:['hello', 'world']
Python中的字符串還有哪些高級用法?
除了上面介紹的常用操作外,Python中的字符串還有許多高級用法,例如:
- 字符串格式化:可以使用字符串的format方法將變量的值插入到字符串中,例如:
name = 'Alice'
age = 20
print('My name is {} and I am {} years old.'.format(name, age))
# 輸出:My name is Alice and I am 20 years old.
- 正則表達式:Python中的re模塊提供了正則表達式的支持,可以方便地進行字符串匹配和替換,例如:
import re
s = 'hello world'
s = re.sub('world', 'python', s)
print(s) # 輸出:hello python
- 字符串編碼:Python中的字符串默認使用Unicode編碼,但是在網絡傳輸和存儲時可能需要進行編碼轉換,例如:
s = '你好'
s = s.encode('utf-8') # 將字符串編碼為utf-8格式的字節串
print(s) # 輸出:b'\xe4\xbd\xa0\xe5\xa5\xbd'
- 字符串加密:Python中的hashlib模塊提供了多種哈希函數,可以用于字符串的加密和校驗,例如:
import hashlib
s = 'hello world'
md5 = hashlib.md5()
md5.update(s.encode('utf-8'))
print(md5.hexdigest()) # 輸出:5eb63bbbe01eeed093cb22bb8f5acdc3
這些高級用法需要一定的編程經驗和知識儲備,但是對于一些復雜的應用場景非常有用。
Python中的st相關問答
1. Python中的字符串是不是可變的?
答:不可變的。一旦創建了一個字符串,就不能修改它的值。
2. Python中如何將字符串轉換為列表?
答:可以使用字符串的split方法將字符串按照某個分隔符分割成多個子串,例如:
s = 'hello,world'
lst = s.split(',')
print(lst) # 輸出:['hello', 'world']
3. Python中如何將字符串轉換為整數?
答:可以使用int函數將字符串轉換為整數,例如:
s = '123'
n = int(s)
print(n) # 輸出:123
4. Python中如何將字符串轉換為浮點數?
答:可以使用float函數將字符串轉換為浮點數,例如:
s = '3.14'
f = float(s)
print(f) # 輸出:3.14
5. Python中如何判斷兩個字符串是否相等?
答:可以使用==運算符判斷兩個字符串是否相等,例如:
s1 = 'hello'
s2 = 'world'
if s1 == s2:
print('equal')
else:
print('not equal')
6. Python中如何將字符串中的所有字母轉換為大寫或小寫?
答:可以使用upper方法將字符串中的所有字母轉換為大寫,也可以使用lower方法將字符串中的所有字母轉換為小寫,例如:
s = 'Hello World'
s = s.upper()
print(s) # 輸出:HELLO WORLD
s = s.lower()
print(s) # 輸出:hello world
7. Python中如何判斷一個字符串是否包含另一個字符串?
答:可以使用in運算符判斷一個字符串是否包含另一個字符串,例如:
s = 'hello world'
if 'world' in s:
print('contains')
else:
print('not contains')
8. Python中如何去除字符串中的空格?
答:可以使用strip方法去除字符串中的空格,例如:
s = ' hello world '
s = s.strip()
print(s) # 輸出:hello world
9. Python中如何判斷一個字符串是否以某個子串開頭或結尾?
答:可以使用startswith方法判斷一個字符串是否以某個子串開頭,也可以使用endswith方法判斷一個字符串是否以某個子串結尾,例如:
s = 'hello world'
if s.startswith('hello'):
print('starts with hello')
else:
print('not starts with hello')
if s.endswith('world'):
print('ends with world')
else:
print('not ends with world')
10. Python中如何將多個字符串拼接成一個字符串?
答:可以使用join方法將多個字符串拼接成一個字符串,例如:
lst = ['hello', 'world']
s = ' '.join(lst)
print(s) # 輸出:hello world
以上就是關于Python中的st的相關介紹和問答,希望對大家有所幫助。

相關推薦HOT
更多>>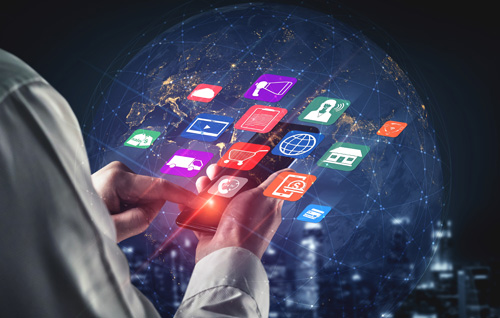
python全注釋快捷鍵
Python全注釋快捷鍵:提高編程效率的利器Python是一種高級編程語言,被廣泛應用于數據分析、人工智能等領域。在Python編程中,注釋是非常重要的...詳情>>
2023-11-17 23:36:31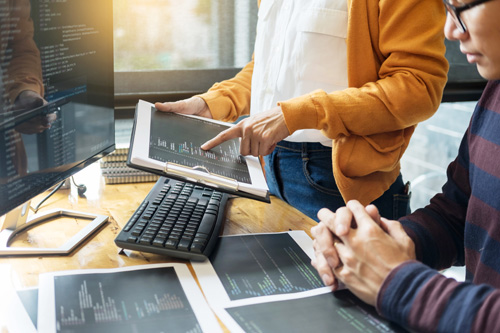
python代碼沒錯但運行不出來,只有一條線
Python代碼沒錯但運行不出來,只有一條線。這可能是每個Python程序員都曾經遇到過的問題。代碼看起來沒有任何語法錯誤,但程序運行時卻只輸出了...詳情>>
2023-11-17 22:22:44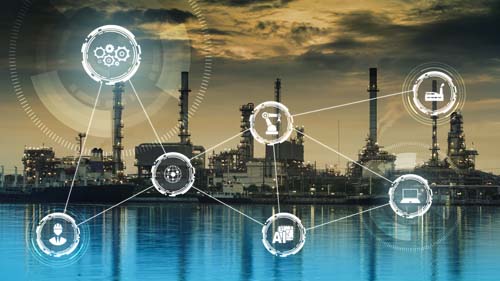
python中兩個等于號
==Python中的兩個等于號==在Python中,我們經常會用到兩個等于號(==),它們代表著什么意思呢?這篇文章將為你詳細解答這個問題,并擴展相關問...詳情>>
2023-11-17 21:20:25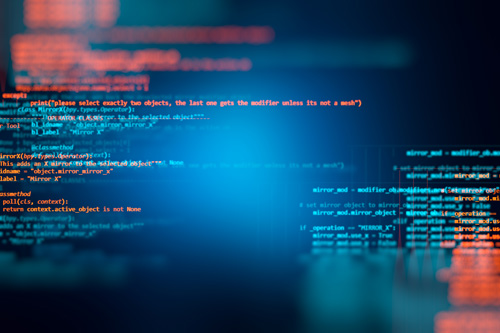
python中len()的用法
Python中的len()函數是一個非常常用的函數,它用于獲取一個序列的長度或者一個字符串的字符數。len()函數接受一個參數,即要獲取長度的序列或字...詳情>>
2023-11-17 19:26:23